5 Ways to Check Excel Sheet Existence in Java

Working with Excel files in Java can be quite common, especially for tasks involving data analysis, reporting, or automation. Before you start manipulating or reading an Excel workbook, it's crucial to verify whether the sheet you intend to work with actually exists. This step ensures that your program can handle errors gracefully or proceed with specific actions if a sheet is missing. In this post, we'll explore five different methods to check if an Excel sheet exists in Java, using different libraries and approaches.
1. Using Apache POI Library
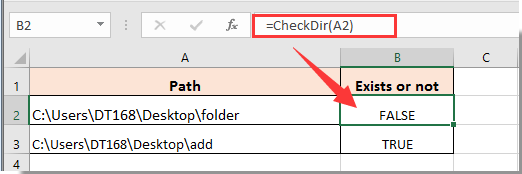
Apache POI is one of the most popular libraries for working with Microsoft Office documents in Java. Here’s how you can check for sheet existence using POI:
- Dependencies: Add Apache POI to your project via Maven or by downloading the jar files.
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public void checkSheetExistsPOI(String filePath, String sheetName) throws IOException {
FileInputStream fis = new FileInputStream(new File(filePath));
Workbook workbook = new XSSFWorkbook(fis);
// Check if sheet exists
Sheet sheet = workbook.getSheet(sheetName);
if (sheet != null) {
System.out.println("The sheet " + sheetName + " exists.");
} else {
System.out.println("The sheet " + sheetName + " does not exist.");
}
workbook.close();
fis.close();
}
Notes
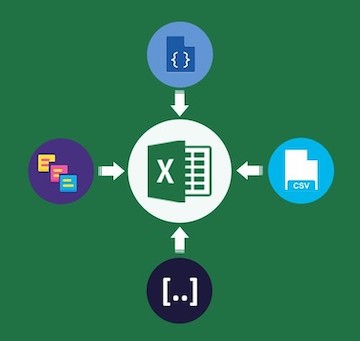
⚠️ Note: This method only checks if the sheet name matches case-insensitively but does not ensure the sheet is not hidden or very hidden.
2. Using JXL (JExcelApi)

JExcelApi, or JXL, is an older library for handling Excel files, which works with .xls files only. Here’s how to use it:
import jxl.Sheet;
import jxl.Workbook;
import jxl.read.biff.BiffException;
import java.io.File;
import java.io.IOException;
public boolean checkSheetExistsJXL(String filePath, String sheetName) throws IOException, BiffException {
Workbook workbook = Workbook.getWorkbook(new File(filePath));
for (Sheet s : workbook.getSheets()) {
if (s.getName().equalsIgnoreCase(sheetName)) {
System.out.println("The sheet " + sheetName + " exists.");
return true;
}
}
System.out.println("The sheet " + sheetName + " does not exist.");
return false;
}
3. Using Stream API with Apache POI

For a more modern Java approach, we can leverage the Stream API along with POI:
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Optional;
public boolean sheetExistsStream(String filePath, String sheetName) throws IOException {
FileInputStream fis = new FileInputStream(new File(filePath));
Workbook workbook = new XSSFWorkbook(fis);
return workbook.sheetIterator()
.anyMatch(sheet -> sheetName.equalsIgnoreCase(sheet.getSheetName()));
}
4. Custom Implementation
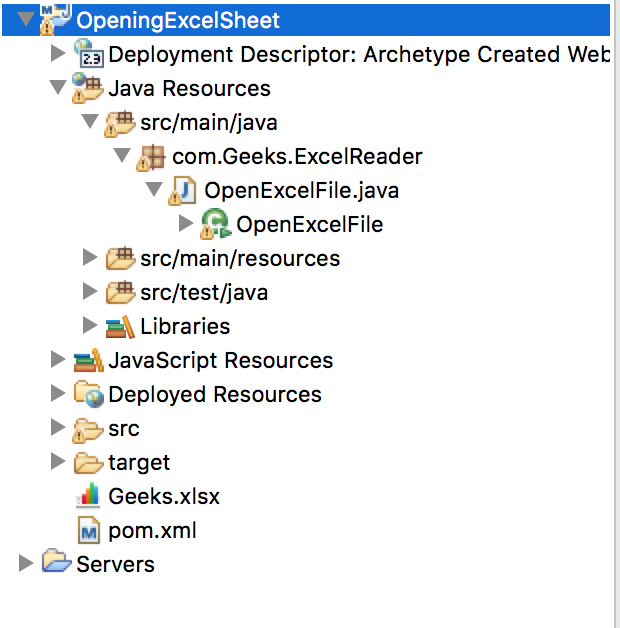
Sometimes, you might not want to rely on external libraries for simple checks. Here’s a basic implementation without using any additional libraries:
import java.io.FileInputStream;
import java.io.IOException;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public boolean customSheetExistsCheck(String filePath, String sheetName) throws IOException {
try (FileInputStream fis = new FileInputStream(filePath);
ZipInputStream zis = new ZipInputStream(fis)) {
ZipEntry entry;
while ((entry = zis.getNextEntry()) != null) {
if (entry.getName().equals("xl/worksheets/sheet" + sheetName.toLowerCase() + ".xml")) {
return true;
}
}
return false;
}
}
📝 Note: This method assumes the .xlsx file structure, which is a zip archive with XML entries for worksheets. This approach might fail if sheets are renamed or if the file is corrupted.
5. Using Java 11 HttpClient for Online Check

If your Excel file is accessible online, you could use Java 11’s HttpClient to check for sheet existence without downloading the entire file:
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.concurrent.CompletableFuture;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public CompletableFuture checkSheetExistsOnline(String url, String sheetName) throws IOException, InterruptedException {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create(url))
.build();
return client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenApply(response -> {
Pattern pattern = Pattern.compile("sheetName=\"" + sheetName + "\"", Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(response.body());
return matcher.find();
});
}
Each method above has its pros and cons regarding dependencies, ease of implementation, and flexibility:
- Apache POI: Versatile, supports many Excel formats, but adds significant overhead.
- JXL: Simple for .xls files, but not updated for newer Excel formats.
- Stream API with POI: Uses modern Java features, but still depends on POI.
- Custom Implementation: Minimal dependency but limited in capabilities.
- Online Check: Useful for remote files, but requires internet connectivity and file format predictability.
In summary, checking for the existence of an Excel sheet in Java can be done through various methods, each suited to different scenarios based on your application's needs, the size of the file, file format, and accessibility of the data. Remember that error handling, file format detection, and checking for hidden sheets are crucial aspects to consider for a robust implementation. Now, let's address some common questions related to this topic:
What is the advantage of using Apache POI over other libraries?

+
Apache POI supports both the old (.xls) and new (.xlsx) Excel formats, offers extensive functionality for Excel manipulation, has good community support, and is regularly updated to handle newer Excel features and security patches.
How do you handle case-sensitivity when checking sheet names?

+
Use equalsIgnoreCase()
or create a pattern with Pattern.CASE_INSENSITIVE
flag in regex matching to ensure case insensitivity.
Can these methods work with hidden sheets?

+
Yes, these methods will detect hidden sheets unless you explicitly check for their visibility status. With Apache POI, you can call isSheetHidden(int sheetIndex)
to check if a sheet is hidden.
Is there a way to check for sheet existence without reading the entire file?

+
The custom implementation reading ZIP entries or the online check via HTTP are examples of such methods where you can check sheet names without loading the entire Excel file into memory.