5 Simple Ways to Rename Excel Sheets with VBA
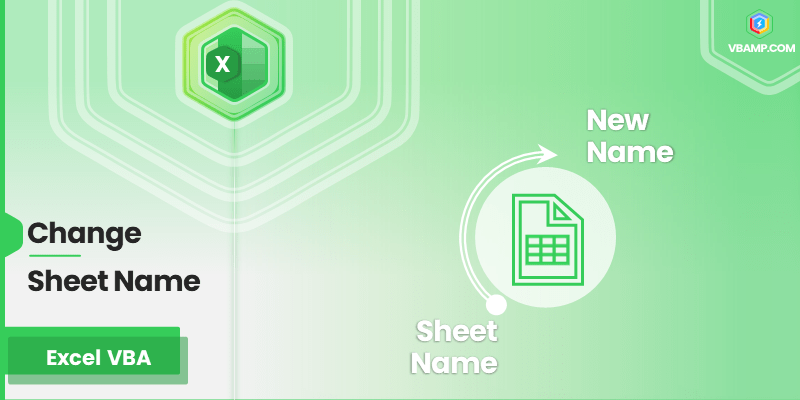
Renaming Excel sheets dynamically can streamline your workflow, enhance user experience, and make your spreadsheets more intuitive and organized. Visual Basic for Applications (VBA) in Excel provides a powerful toolset to automate repetitive tasks, including the renaming of worksheet tabs. Here are five simple ways to use VBA for renaming your Excel sheets, helping you achieve a more structured spreadsheet setup.
Using the Worksheet’s Name Property Directly


The most straightforward method involves changing the Name property of a worksheet. Here’s how you can do it:
- Press Alt + F11 to open the VBA editor.
- Insert a new module by clicking Insert > Module.
- Paste the following code into your module:
Sub RenameActiveSheet()
ActiveSheet.Name = “NewSheetName”
End Sub
When you run this macro, it will rename the active sheet to “NewSheetName”. You can change “NewSheetName” to any name you prefer.
Batch Rename Using a Loop

If you have multiple sheets to rename, a loop can be very handy. Here’s how you can batch rename:
- Use a For…Next loop to cycle through all sheets:
Sub BatchRenameSheets() Dim ws As Worksheet Dim newName As String Dim i As Integer
i = 1 For Each ws In ThisWorkbook.Sheets newName = "Sheet" & i ws.Name = newName i = i + 1 Next ws
End Sub
This code will rename each sheet sequentially, beginning with “Sheet1”, “Sheet2”, etc.
Renaming Sheets Based on Cell Content
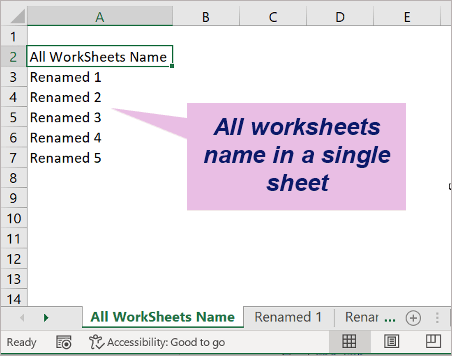
Sometimes, the new name for the sheet might be determined by data within the sheet itself:
- Use this code to rename based on content from a specific cell:
Sub RenameBasedOnCell() Dim ws As Worksheet Dim cellValue As String
Set ws = ActiveSheet cellValue = ws.Range("A1").Value ' Assuming cell A1 contains the name ws.Name = cellValue
End Sub
⚠️ Note: Ensure the value in A1 is valid as a sheet name (doesn’t contain invalid characters, isn’t too long, and isn’t already in use).
Event-Driven Sheet Renaming

Instead of calling a macro manually, you can automate the renaming process based on events. For instance, renaming the sheet every time data in a specific cell changes:
- Add the following to the Worksheet module for the sheet you want to monitor:
Private Sub Worksheet_Change(ByVal Target As Range)
If Not Intersect(Target, Me.Range(“A1”)) Is Nothing Then
Me.Name = Me.Range(“A1”).Value
End If
End Sub
Using InputBox for Interactive Renaming
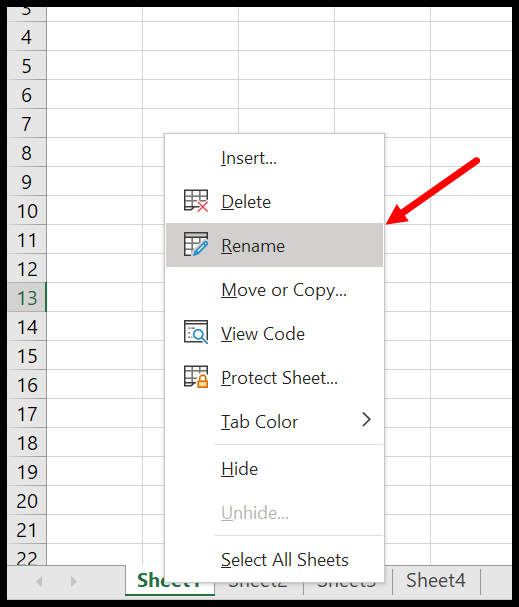
If you want users to have a say in the renaming process, an InputBox can be quite useful:
Sub InteractiveRename() Dim newName As String Dim ws As Worksheet
Set ws = ActiveSheet newName = InputBox("Enter the new name for the sheet:") If newName = "" Then MsgBox "No name entered. Rename cancelled." Else ws.Name = newName End If
End Sub
Here, the user is prompted to type in the new name for the active sheet.
These methods of renaming sheets in Excel using VBA not only save time but also make data management within Excel more dynamic and user-friendly. By leveraging VBA, you can rename sheets automatically, interactively, or based on specific events or conditions, which can greatly enhance the functionality and usability of your spreadsheets.
Can I undo a VBA rename operation?
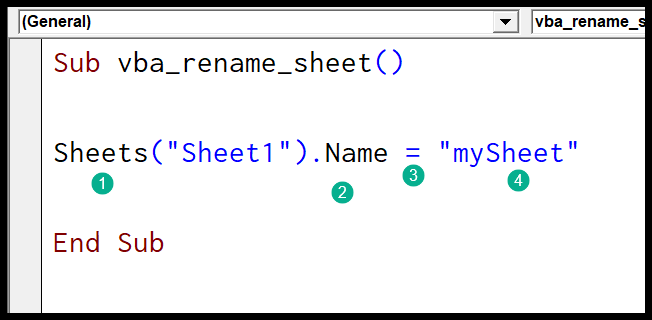
+
Unlike standard Excel actions, VBA macros do not have an automatic undo feature. You would need to manually revert the changes or keep a backup of your original sheet names before running the macro.
Is there a limit to the number of times I can rename a sheet with VBA?

+
There’s no hard limit, but excessive renaming can be computationally intensive. Always check for efficiency in your macro if it involves multiple operations.
What are the restrictions on sheet names in Excel?

+
Sheet names cannot:
- Contain more than 31 characters
- Contain the following characters: \ / ? * : [ ]
- Be completely blank
- Start or end with a single quote (‘) character