5 Ways to Assign Macros to Entire Excel Sheets
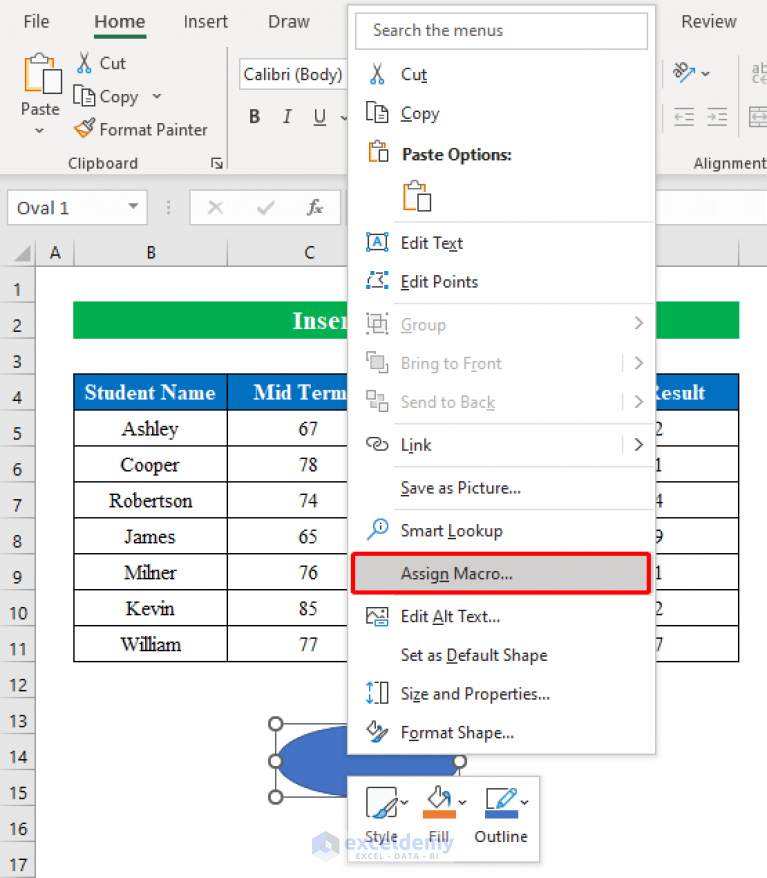
In the realm of Excel macros, efficiency and automation are the watchwords. A key skill to master in this environment is learning how to assign macros to entire Excel sheets, which can drastically reduce the time spent on repetitive tasks. Whether you're dealing with data analysis, financial modeling, or daily administrative tasks, macros can help streamline your workflow. Let's explore five comprehensive methods to apply macros across whole sheets in Excel:
1. Using the Workbook_Open Event

This method leverages the Workbook_Open
event, which automatically runs a macro when an Excel workbook is opened. Here’s how to set it up:
- Open the VBA Editor: Press Alt + F11 to open the Visual Basic for Applications editor.
- Access the Workbook Object: In the Project Explorer, double-click on ‘ThisWorkbook’.
- Add Code: Enter the following code:
Private Sub Workbook_Open()
Call RunMyMacro
End Sub
Now, whenever the workbook is opened, the macro RunMyMacro
will be executed. This approach is particularly useful for initialization or setting up environments automatically.
2. Attaching Macros to Worksheet Events

If your goal is to have a macro execute when specific actions are taken on a worksheet, you can use worksheet events. For instance, to run a macro whenever the worksheet is activated:
- Open the VBA Editor: Use Alt + F11 to get there.
- Select the Worksheet: Double-click the desired worksheet in the Project Explorer.
- Write the Event Code: Add the following event handler:
Private Sub Worksheet_Activate()
Call YourMacroHere
End Sub
💡 Note: Remember to replace YourMacroHere
with the actual name of your macro.
3. Using Macros in Auto_Close Event

This approach runs macros when the workbook is about to be closed, ensuring clean-up or save operations:
- Access 'ThisWorkbook': Double-click 'ThisWorkbook' in the VBA editor.
- Add the Auto_Close event: Use this code:
Private Sub Workbook_BeforeClose(Cancel As Boolean)
Call PerformClosingTasks
End Sub
4. Macros as Sheet Functions

This technique allows you to call macros within worksheet cells:
- Define Function in VBA: In the VBA editor, insert a new module and define a function:
Function MyMacroFunction(x As Variant) As Variant
'Macro logic here
MyMacroFunction = x * 2 ' Example operation
End Function
- Use Function in Cells: In your worksheet, simply enter
=MyMacroFunction(A1)
to use the macro.
⚠️ Note: Macros used as functions should be lightweight to avoid performance issues.
5. Automating Tasks with the Worksheet_Change Event

To make your macro run automatically when data is modified in a specific range:
- Select the Worksheet: Double-click the worksheet in the VBA editor.
- Add the Event Code: Here's an example:
Private Sub Worksheet_Change(ByVal Target As Range)
If Not Intersect(Target, Range("A1:A10")) Is Nothing Then
Call RunSpecificMacro
End If
End Sub
This method is particularly useful for real-time data processing or validation.
In summary, mastering these five methods for assigning macros to entire Excel sheets can significantly boost your productivity. From executing macros on workbook opening or closing to dynamically responding to changes in data, these techniques offer flexible solutions for automating your Excel tasks. Whether you're automating financial reports, managing databases, or performing complex calculations, these approaches help make Excel work smarter for you. Remember to tailor each method to your specific needs and ensure your macros are efficient and error-free for a seamless user experience.
Can macros affect Excel’s performance?
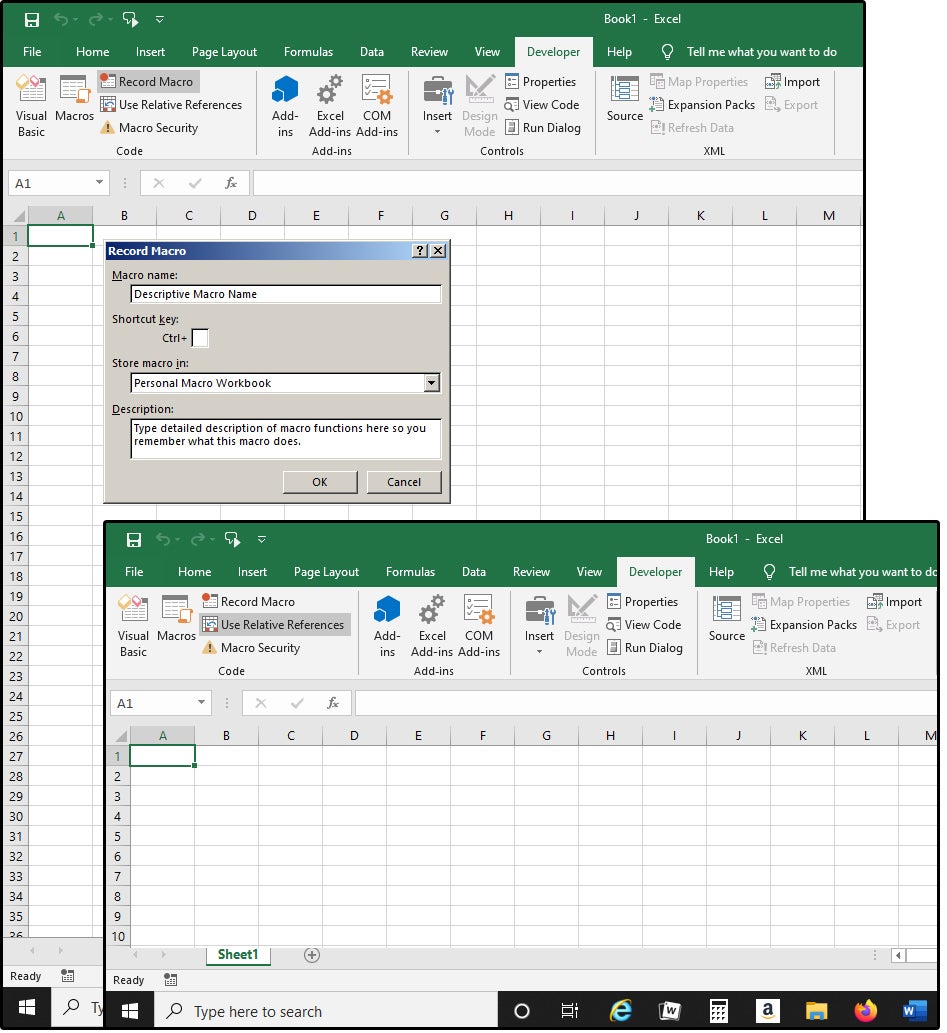
+
Yes, complex macros can slow down Excel’s performance, especially if they involve loops or extensive computations. However, with proper coding practices like disabling screen updating and optimizing loops, the impact can be minimized.
How can I stop macros from running on specific sheets?

+
By checking the sheet’s name or index within your macro’s code, you can add conditional statements to ensure the macro only runs on the intended sheets.
What if I accidentally overwrite important data?

+
Always create backups before running macros that modify data. Additionally, you can implement safeguards within your macros, like prompt confirmations or undo functions.
How do I share macros in my team environment?

+
Macros can be shared by exporting the VBA modules or the entire workbook, or by placing common macros in the ‘Personal.xlsb’ file which is shared across all workbooks for a specific user.
Can macros be used with external data sources?

+
Yes, macros can interact with external databases, files, or online data sources through the use of appropriate VBA libraries and APIs.