5 Python Tips to Append Data in Excel Sheets

The need for programmatically appending data to Excel spreadsheets is common for many who work with data analysis, reporting, or project management. Python, with its versatile libraries like Pandas and openpyxl, makes this task easier than ever. Here are five useful tips to improve your efficiency and accuracy when appending data to Excel sheets using Python.
1. Use Openpyxl for Excel File Manipulation

Openpyxl is an excellent library for working with Excel files in Python. Unlike other libraries that might require an active Excel installation, openpyxl can read, write, and modify Excel files directly from memory. Here’s how you can start:
- Install openpyxl using pip:
pip install openpyxl
- Load an existing workbook with
openpyxl.load_workbook(filename)
.
After loading the workbook:
from openpyxl import load_workbook wb = load_workbook(‘yourfile.xlsx’) sheet = wb.active
sheet.append([‘New’, ‘Data’, ‘Here’]) wb.save(‘yourfile.xlsx’)
📝 Note: Be cautious when appending data to ensure you do not overwrite existing data or create confusion by mixing data types or headers.
2. Leverage Pandas for Batch Data Append
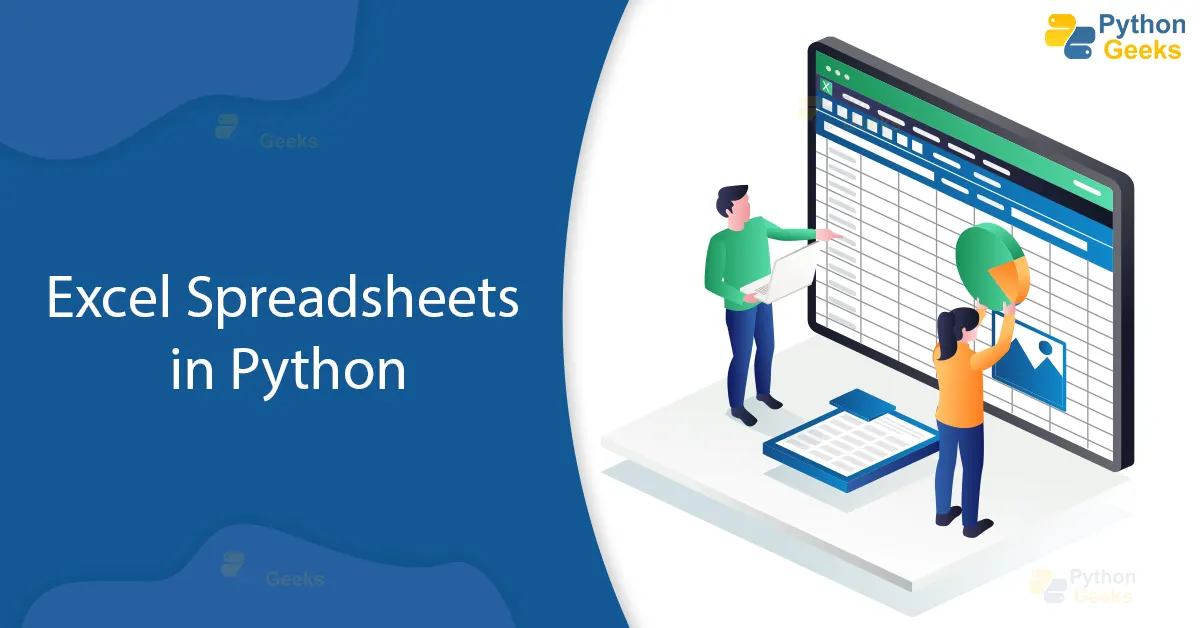
Pandas, the popular data manipulation library in Python, can also help with appending data to Excel, particularly when dealing with large datasets. Here’s how:
- Import Pandas with
import pandas as pd
. - Read or create your DataFrame. Append new data with:
df = pd.read_excel(‘existing_data.xlsx’)
new_data = {‘Column1’: [10, 20], ‘Column2’: [‘text’, ‘moretext’]}
new_df = pd.DataFrame(new_data)
df = pd.concat([df, new_df], ignore_index=True)
df.to_excel(‘existing_data.xlsx’, index=False)
📝 Note: Pandas will overwrite the file unless you specify an 'append' mode or create a temporary file. It's also essential to ensure the data types in your DataFrame align with what already exists in the Excel file.
3. Automate Headers with Openpyxl

When appending new data to an Excel sheet, you might want to add headers automatically if they don’t already exist. Openpyxl makes this straightforward:
- Check if headers exist by looking for specific cells or using cell values:
headers = [‘Header1’, ‘Header2’, ‘Header3’]
if not all(cell.value == header for cell, header in zip(sheet[1], headers)):
for i, header in enumerate(headers, start=1):
sheet.cell(row=1, column=i, value=header)
📝 Note: Always ensure you're not duplicating headers when adding them. Checking for headers can also help prevent overwriting data inadvertently.
4. Organize Data with Multiple Sheets

When dealing with complex datasets, utilizing multiple sheets can keep your data organized. Here’s how to create or access different sheets:
- Create or access a sheet:
if ‘NewSheet’ not in wb.sheetnames: wb.create_sheet(‘NewSheet’) sheet = wb[‘NewSheet’] sheet.append([‘This’, ‘is’, ‘new’, ‘data’]) wb.save(‘yourfile.xlsx’)
📝 Note: When working with multiple sheets, it's good practice to check for their existence to avoid duplicating efforts or overwriting data.
5. Handle Large Datasets Efficiently

When dealing with big data, memory can become a constraint. Here are some strategies:
- Use chunk reading to append data in smaller, manageable sizes:
import pandas as pd
for chunk in chunker: chunk.to_excel(‘yourfile.xlsx’, mode=‘a’, index=False, header=False)
Alternatively, when using openpyxl:
from openpyxl.utils.dataframe import dataframe_to_rows
for chunk in chunker: for row in dataframe_to_rows(chunk, index=False, header=False): sheet.append(row) wb.save(‘yourfile.xlsx’)
📝 Note: Be mindful of Excel's row limits and the performance hit that large data operations can incur. Consider segmenting data into different files if necessary.
Appending data to Excel files programmatically can streamline your data management tasks. Python, combined with libraries like openpyxl and Pandas, provides powerful tools to manage Excel data with ease. By following these tips, you'll ensure data integrity, enhance productivity, and make appending data to Excel sheets a more straightforward process. Incorporate these practices into your workflow to handle data efficiently, even when dealing with large datasets or complex file structures.
Can I append data to an Excel file without overwriting existing data?
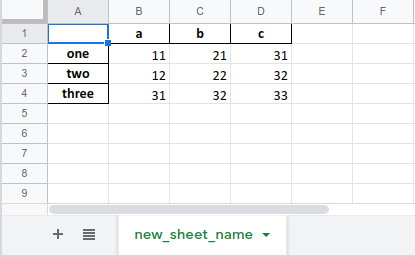
+
Yes, using libraries like Pandas with the append mode or openpyxl by manipulating the workbook in memory, you can add data without overwriting existing data. Ensure to append data to the correct cell or row to avoid conflicts.
How can I handle different data types in Excel when appending with Python?

+
Python libraries like openpyxl can handle different data types by converting them to the appropriate Excel data type. You can also format cells using styles to specify number formats or data types like dates, currency, etc.
What is the best way to append data to an Excel file if the data source changes frequently?

+
Automate the process with a script. Use a cron job or scheduled task to run your Python script at regular intervals to update or append data from sources like databases or APIs to your Excel file.