5 Ways to Access Excel Sheets in Python

In today's data-driven world, the ability to automate tasks and analyze data efficiently is invaluable. Python, known for its versatility in handling data manipulation and analysis tasks, offers several powerful libraries that can interact with Microsoft Excel files. In this blog post, we'll explore five robust methods to access and manipulate Excel sheets using Python, each suited for different needs and levels of complexity. Whether you're a data scientist, an analyst, or just someone looking to streamline your workflow, mastering these techniques will significantly enhance your productivity.
Python Excel Library: openpyxl

Openpyxl is an open-source library specifically designed to read, write, and modify Excel 2010 xlsx/xlsm/xltx/xltm files without involving Microsoft Excel. It's one of the most popular choices due to its ease of use and comprehensive features.
- Installation: First, you need to install openpyxl. Run the command:
pip install openpyxl
- Usage: Here’s how you can read and modify an Excel sheet:
from openpyxl import load_workbook
# Load an existing workbook
wb = load_workbook(filename='example.xlsx')
# Access a specific sheet by name
sheet = wb['Sheet1']
# Reading cell values
print(sheet['A1'].value)
# Writing to a cell
sheet['A1'] = 'New Value'
# Saving the changes
wb.save('example_modified.xlsx')
🚀 Note: Openpyxl works exclusively with the .xlsx format and cannot open .xls files directly.
Python Excel Library: pandas

Pandas is not just for data manipulation; it also excels (pun intended) in reading Excel files with its intuitive DataFrame structure.
- Installation: Install pandas with
pip install pandas openpyxl
(you need openpyxl for Excel I/O). - Usage: Here’s how to read and manipulate Excel data with pandas:
import pandas as pd
# Read the Excel file
df = pd.read_excel('example.xlsx', sheet_name='Sheet1')
# Display the first few rows
print(df.head())
# Perform operations on DataFrame (e.g., filtering)
filtered_data = df[df['column_name'] > 50]
# Write to a new Excel file
filtered_data.to_excel('filtered_data.xlsx', index=False)
Python Excel Library: xlrd

If you need to work with older Excel file formats (.xls), xlrd is your library of choice, offering backward compatibility for reading Excel 97-2003 .xls files.
- Installation: Simply run
pip install xlrd
- Usage: Here’s a basic example:
import xlrd
# Open the workbook
book = xlrd.open_workbook('example.xls')
# Select the first sheet
sheet = book.sheet_by_index(0)
# Access cell values
print(sheet.cell_value(0, 0))
📚 Note: Although pandas can read .xls files via xlrd, the latter is more suited for older Excel versions.
Python Excel Library: pyexcel

Pyexcel is an abstraction layer that simplifies handling various file formats, making it versatile for file reading and writing.
- Installation: Install it using
pip install pyexcel pyexcel-xlsx
(for .xlsx support). - Usage: Here’s how you can use pyexcel:
import pyexcel as pe
# Read from an Excel file
data = pe.get_array(file_name='example.xlsx')
# Writing back to an Excel file
pe.save_as(array=data, dest_file_name='example_new.xlsx')
Python Excel Library: win32com

For those who need to interact with Microsoft Excel directly, perhaps to utilize Excel's own capabilities, win32com provides an interface to automate Excel through COM objects.
- Installation: Install
pip install pywin32
- Usage: Here's how you can open an Excel file:
from win32com.client import Dispatch
# Dispatch the Excel application
xl = Dispatch('Excel.Application')
# Open a workbook
wb = xl.Workbooks.Open('example.xlsx')
# Activate the first sheet
sheet = wb.Sheets('Sheet1')
# Read value from cell A1
print(sheet.Cells(1, 1).Value)
# Close and save
wb.SaveAs('example_modified.xlsx')
wb.Close()
xl.Quit()
In summary, Python offers a variety of tools for Excel manipulation, each with its strengths:
- openpyxl for direct, programmatic access to modern Excel files.
- pandas for data manipulation and analysis within Excel.
- xlrd for compatibility with older Excel formats.
- pyexcel for a simple interface across various file formats.
- win32com for full automation of Excel, though limited to Windows environments.
Each method has its niche:
- Developers and data analysts might prefer openpyxl or pandas for their ease of integration with Python workflows and rich feature sets.
- Those dealing with legacy data or needing to read older files might choose xlrd.
- Pyexcel serves as a versatile tool for quick file operations.
- Win32com is for those who require Excel's functionalities directly.
Choose the method that best aligns with your project requirements, system constraints, and level of Excel integration needed. By mastering these tools, you'll unlock new levels of efficiency in handling Excel data in Python, allowing you to focus more on analysis and less on data retrieval.
Can Python read any version of Excel files?
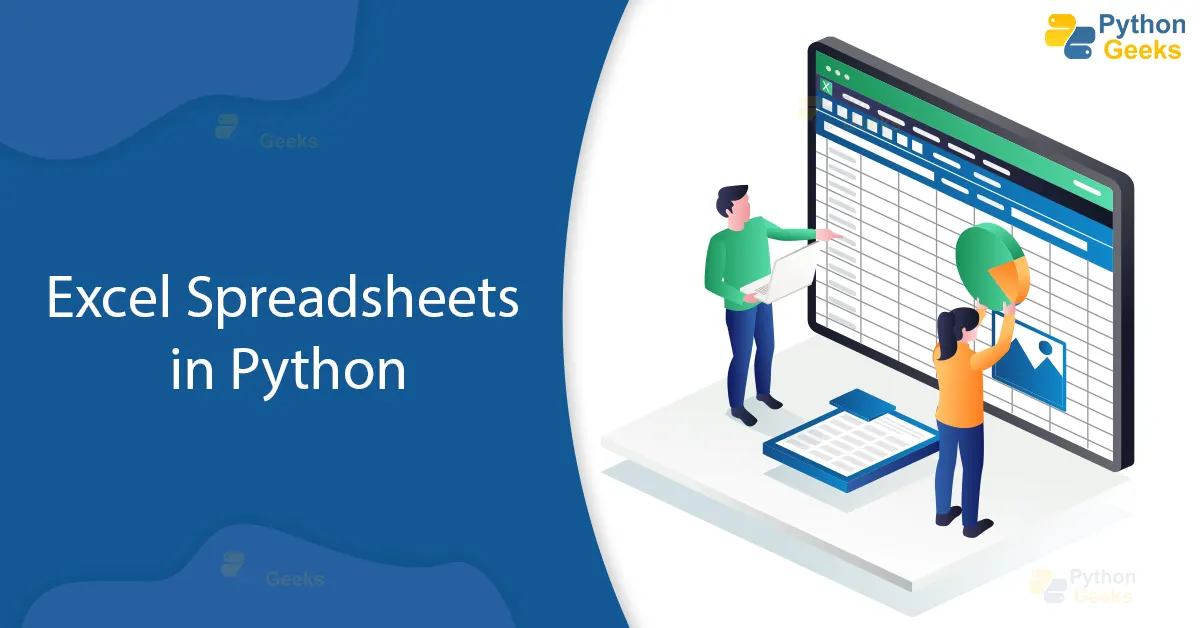
+
Python libraries like openpyxl and pandas primarily work with the .xlsx format. For older .xls files, xlrd is preferred. However, some libraries, like pandas, can use xlrd behind the scenes to handle .xls files, providing a unified interface for both.
What are the system requirements for win32com?

+
win32com requires Windows as it interacts with the Microsoft COM framework to automate Excel, making it platform-specific.
How can I update Excel files without having Excel installed?

+
Libraries like openpyxl, pandas, and pyexcel do not require Excel to be installed. They manipulate the file structure directly, making them ideal for scenarios where Excel is not available.