5 Ways to Access Excel Data in Java Easily
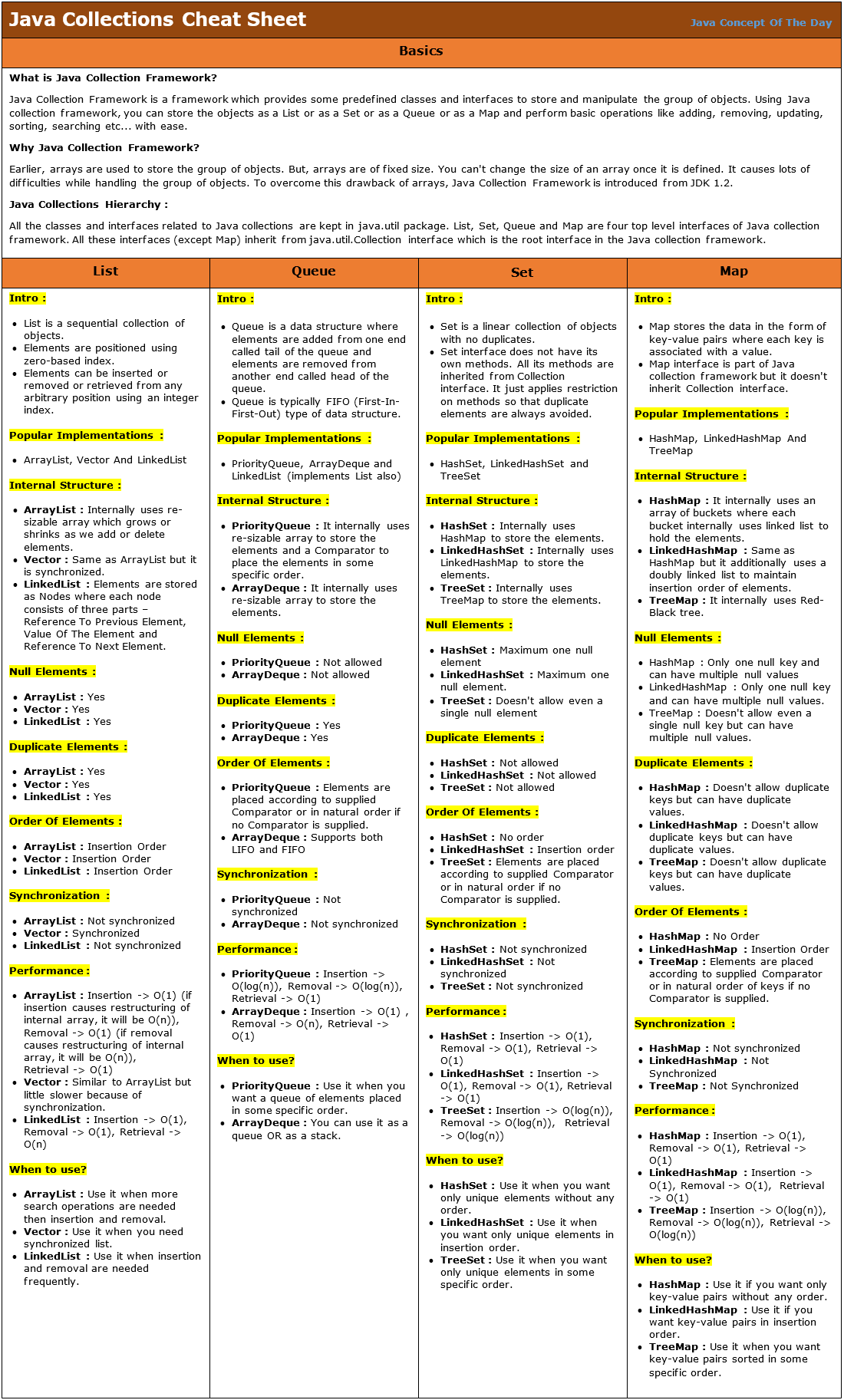
Java developers often find themselves needing to work with Excel files to analyze, process, and manipulate data efficiently. Whether it's for importing, exporting, or processing complex datasets, accessing Excel data can be a crucial part of a Java-based application. Here are five straightforward methods to achieve this:
1. Apache POI

Apache POI is one of the most popular libraries for working with Microsoft Office documents, especially Excel. It offers comprehensive support for both .xls (Excel 97-2003) and .xlsx (Excel 2007 onwards) files.
- Adding POI Dependencies:
- Add POI to your project through a dependency management tool like Maven or Gradle.
- Reading Excel Files:
- Use the HSSF (Horrible SpreadSheet Format) for .xls files.
- Use XSSF (XML SpreadSheet Format) for .xlsx files.
- Writing to Excel Files:
- Create or modify worksheets, rows, and cells programmatically.
- Example Code:
import org.apache.poi.ss.usermodel.*; import org.apache.poi.xssf.usermodel.XSSFWorkbook; public void readExcelFile(String filePath) throws IOException { FileInputStream fileInputStream = new FileInputStream(new File(filePath)); Workbook workbook = new XSSFWorkbook(fileInputStream); Sheet sheet = workbook.getSheetAt(0); Iterator
rowIterator = sheet.iterator(); while (rowIterator.hasNext()) { Row row = rowIterator.next(); Iterator
cellIterator = row.cellIterator(); while (cellIterator.hasNext()) { Cell cell = cellIterator.next(); switch (cell.getCellType()) { case STRING: System.out.print(cell.getStringCellValue() + "\t"); break; case NUMERIC: System.out.print(cell.getNumericCellValue() + "\t"); break; // ... other cell types } } System.out.println(); } workbook.close(); fileInputStream.close(); } |
📝 Note: Make sure to handle the exception correctly by wrapping the code in a try-catch block or letting the exceptions bubble up to a higher level handler.
2. JExcelApi

JExcelApi is another lightweight library used specifically for dealing with Excel files in Java, focusing mainly on .xls format.
- Importing JExcelApi:
- Add the JExcelApi library to your project.
- Basic Reading:
- Read cell data using the Workbook and Sheet classes.
- Writing Data:
- Modify or create new workbooks, sheets, and cells.
- Example Code:
import jxl.*; public void readExcelFile(String filePath) throws IOException, BiffException { Workbook workbook = Workbook.getWorkbook(new File(filePath)); Sheet sheet = workbook.getSheet(0); for (int i = 0; i < sheet.getRows(); i++) { for (int j = 0; j < sheet.getColumns(); j++) { Cell cell = sheet.getCell(j, i); System.out.print(cell.getContents() + "\t"); } System.out.println(); } workbook.close(); }
3. Java Excel API (JXL)

JXL, or Java Excel API, is similar to JExcelApi in functionality but offers additional features like formatting.
- Getting Started with JXL:
- Include JXL in your project dependencies.
- Features:
- Can handle data, formatting, and chart information.
- Example Code:
import jxl.write.*; import jxl.*; public void writeExcelFile(String filePath, String data) throws IOException, WriteException { WritableWorkbook workbook = Workbook.createWorkbook(new File(filePath)); WritableSheet sheet = workbook.createSheet("Data", 0); Label label = new Label(0, 0, data); sheet.addCell(label); workbook.write(); workbook.close(); }
4. OpenCSV

While OpenCSV doesn't directly work with Excel files, it's invaluable for handling CSV files, which can easily be converted to or from Excel data.
- Handling CSV:
- Use OpenCSV to read from or write to CSV files, which can be manually or programmatically converted to Excel or vice versa.
- Example Code:
import com.opencsv.CSVReader; public void readCsvFile(String filePath) throws IOException { CSVReader reader = new CSVReader(new FileReader(filePath)); String[] nextLine; while ((nextLine = reader.readNext()) != null) { for (String string : nextLine) { System.out.print(string + "\t"); } System.out.println(); } reader.close(); }
5. JDB (JDB Framework)

JDB is a versatile framework that can handle Excel files among other formats, providing a high-level abstraction for accessing and manipulating data.
- Setting Up JDB:
- Incorporate JDB into your project's classpath.
- Features:
- Read and write multiple file formats including Excel.
- Example Code:
import net.sf.jdb.*; public void readExcelFile(String filePath) throws Exception { JDBImportReader reader = new JDBImportReader(filePath); while (reader.readNext()) { String data = reader.get(0); System.out.print(data + "\t"); } reader.close(); }
Each of these methods has its strengths and best use cases:
- Apache POI for comprehensive support of Excel features.
- JExcelApi for lightweight operations with older Excel formats.
- JXL for slightly more advanced functionality than JExcelApi.
- OpenCSV for CSV handling, useful when conversion between formats is involved.
- JDB for a unified approach to different file formats.
The choice depends on the specific requirements of your project, the type of Excel file you're dealing with, and the level of interaction you need with Excel's features. By understanding these options, you can select the most suitable method for your Java application, ensuring efficient and effective data processing.
Why do developers prefer Apache POI over other libraries?

+
Apache POI provides extensive support for Excel features, making it suitable for complex data manipulation tasks and is well-maintained with a large user community.
Can I use OpenCSV for Excel files?

+
OpenCSV isn’t meant for Excel directly but can be used in conjunction with tools that convert between CSV and Excel formats, offering a lightweight solution for basic data handling.
What is the benefit of using JExcelApi?

+
JExcelApi is lightweight and performs well for simple data reading from older Excel files (.xls), though it lacks the extensive feature set of Apache POI.