Access Excel Sheets in R: Easy Guide
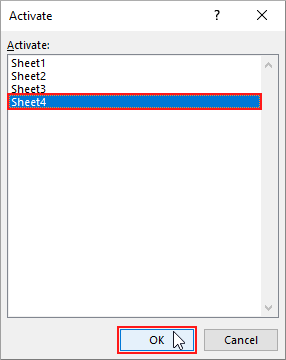
Welcome to our comprehensive guide on how to access Excel sheets in R. R, a powerful tool for statistical computing and graphics, offers various packages that facilitate interaction with Excel files. This post will walk you through the steps to integrate Excel with R, helping you to analyze your data with ease.
Why Use R with Excel?

Before diving into the technical details, let's understand why you might want to use R with Excel:
- Data Cleaning and Preparation: R offers advanced tools for data cleaning and preprocessing, which can be cumbersome with Excel's manual processes.
- Complex Analyses: Excel might struggle with complex statistical or machine learning tasks, which R can handle efficiently.
- Automation and Efficiency: Automating routine tasks saves time, especially when dealing with large datasets or repetitive analyses.
- Reproducibility: R scripts allow for reproducibility, ensuring your work can be verified or replicated by others.
Prerequisites
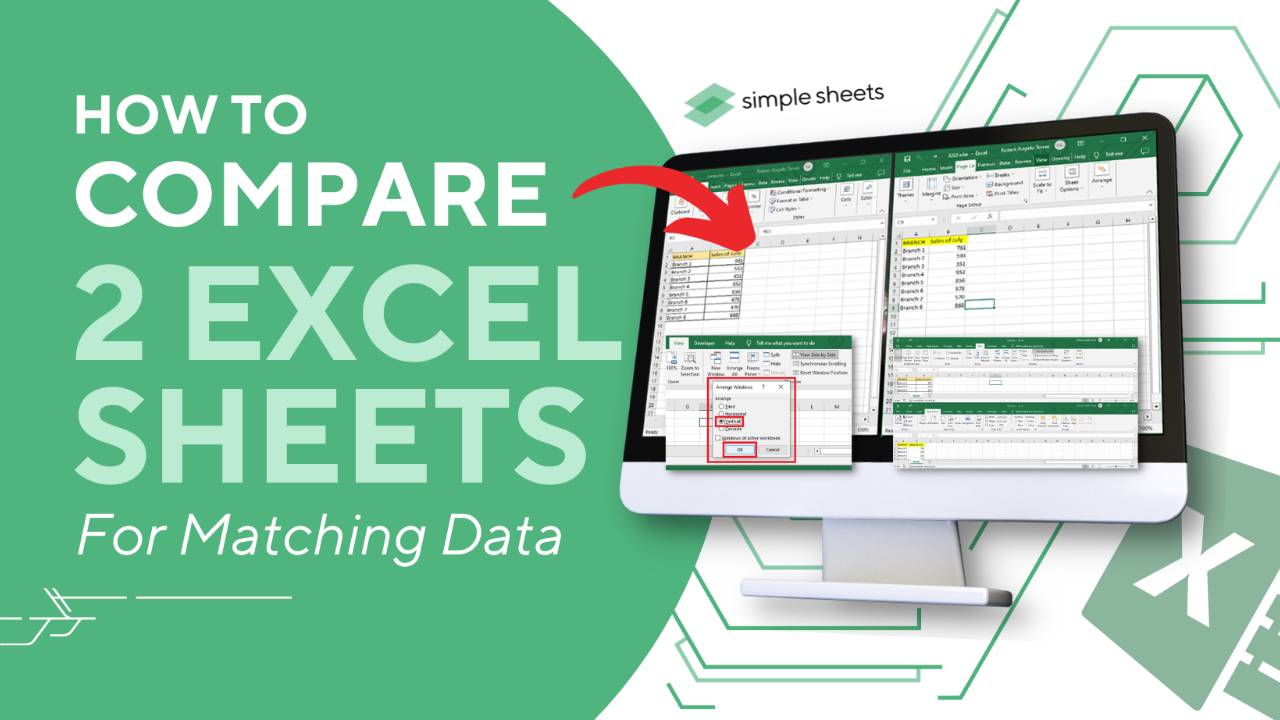
Here’s what you need before you begin:
- An installation of R and RStudio.
- An Excel file (.xls or .xlsx) with data you want to work with.
readxl
package installed in R, which you can do by runninginstall.packages("readxl")
.
Reading Excel Files

Let's get started with importing data from Excel into R:
Using readxl

library(readxl)
# Read the first sheet of an Excel file
data <- read_excel("path/to/yourfile.xlsx")
# Read a specific sheet by name
data_sheet2 <- read_excel("path/to/yourfile.xlsx", sheet = "Sheet2")
💡 Note: The `readxl` package requires the file path to be enclosed in quotes.
Handling Multiple Sheets

If your Excel workbook has multiple sheets, you might want to read them all or select specific ones:
# Get a list of all sheet names
sheet_names <- excel_sheets("path/to/yourfile.xlsx")
# Read all sheets into a list of data frames
data_list <- lapply(sheet_names, read_excel, path = "path/to/yourfile.xlsx")
# Name the list elements with sheet names for easier reference
names(data_list) <- sheet_names
🔍 Note: Using `lapply` creates a list where each element corresponds to a data frame from each sheet.
Specifying Ranges

You can specify a range to import:
data_range <- read_excel("path/to/yourfile.xlsx", range = "A1:C10")
Writing to Excel Files

Exporting data from R to Excel can be achieved with the writexl
package:
install.packages("writexl")
library(writexl)
write_xlsx(data, "path/to/output.xlsx")
Exporting Multiple Data Frames

If you need to export multiple data frames into different sheets of the same Excel file:
write_xlsx(list(data1 = df1, data2 = df2, data3 = df3), "path/to/multiple_sheets.xlsx")
📝 Note: The list's names become the sheet names in the resulting Excel file.
Advanced Techniques

Here are some advanced tips for working with Excel in R:
Data Modification

After importing data, you might want to modify it before analysis:
- Change column types, e.g., convert date strings to proper date format.
- Replace NA values.
- Filter or subset data.
data$Date <- as.Date(data$Date)
data[is.na(data)] <- 0
filtered_data <- data[data$Column > 50, ]
Handling Formulas

While R can read Excel formulas, they are imported as their calculated values. If you need to preserve or work with formulas, consider using the openxlsx
package:
library(openxlsx)
# Preserve formulas when writing data back to Excel
write.xlsx(data_with_formulas, "path/to/output.xlsx", overwrite = TRUE)
Conclusion

Integrating Excel with R provides a robust solution for data manipulation and analysis. You can seamlessly import Excel sheets into R, perform complex analyses, and then export the results back into Excel for presentation or further processing. This guide has equipped you with the basic and advanced methods to leverage both R's analytical power and Excel's widespread use in data reporting and visualization.
How do I install packages in R?

+
You can install packages in R using the install.packages()
function. For example, to install the readxl
package, you would use install.packages(“readxl”)
in your R console.
Can I read password-protected Excel files in R?

+
Standard packages like readxl
do not support reading password-protected Excel files directly. However, you might consider using external tools to remove the password or explore packages like RODBC
or RExcel
that might offer such functionality.
What should I do if I encounter encoding issues with Excel files?
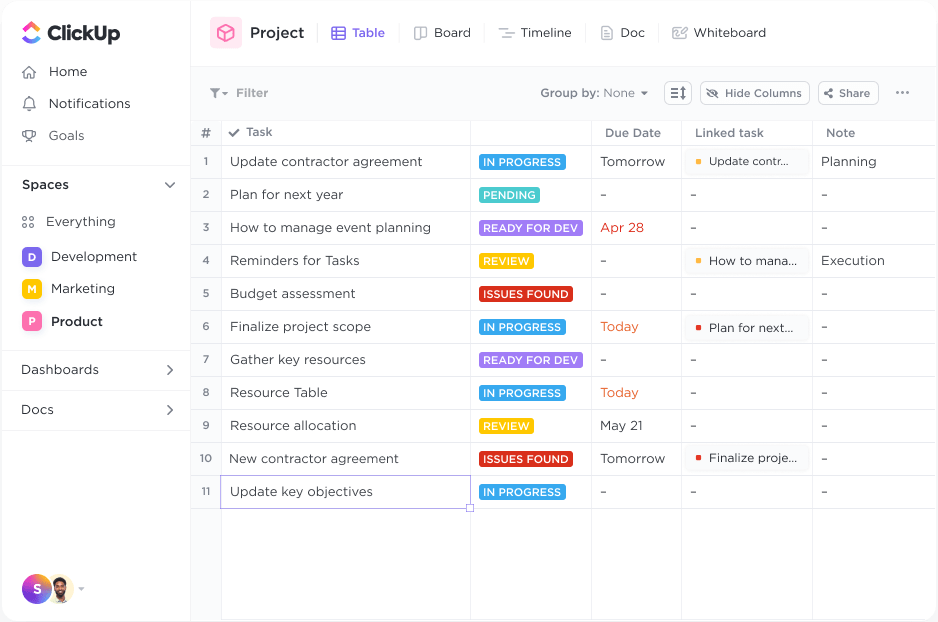
+
Encoding problems can often be resolved by specifying the correct encoding when reading the file, such as read_excel(path, encoding = “UTF-8”)
. Check the encoding used in the Excel file or try different common encodings until you find the one that works.