Understanding C Naming Conventions in Excel Sheets

An Overview of C Naming Conventions

Naming conventions play a crucial role in programming. They enhance readability, maintainability, and the overall understanding of the code for both the developer and future reviewers. In the world of C programming, certain naming conventions have become standard practices. Let's explore the commonly accepted naming conventions for variables, functions, constants, and macros in C.
Variables

Variables in C should follow these guidelines:
- Use lowercase letters for the first word and capitalize the first letter of subsequent words (camelCase).
- Choose names that indicate the variable's purpose or value.
- Avoid abbreviations unless they are widely recognized or standardized in the codebase.
Example:
int userDataCount;
⚠️ Note: For local variables within functions, shorter names might be acceptable for brevity, but still use meaningful names.
Functions

Function names in C typically:
- Follow camelCase or use underscores to separate words.
- Use verbs to describe the action the function performs.
Example:
void calculateTotalSum();
Constants and Macros

Constants and macros should:
- Use all uppercase letters with underscores to separate words.
- Be descriptive of their purpose or the value they represent.
Example:
#define MAX_BUFFER_SIZE 1024
Global Variables

While it's often recommended to avoid global variables, when necessary:
- Prefix them with a 'g' or another distinctive character to clearly identify them as global.
- Follow camelCase or underscore notation.
Example:
int gUserCount;
Type Definitions

For type definitions using typedef, consider:
- Using a lowercase letter for the first word followed by capitalized words (e.g., camelCase).
- Prefixing with the existing type name can provide context.
Example:
typedef struct user_data UserData;
Structures

Structures in C naming conventions usually:
- Use capital letters for the first letter of each word (PascalCase).
Example:
struct UserData {
int count;
char name[30];
};
Arrays

Naming conventions for arrays should:
- Indicate the purpose or content of the array.
- Use singular names for elements and plural for arrays, where applicable.
Example:
int itemIndex;
// singular for an element
int items[100];
// plural for array
Integrating C Naming Conventions in Excel

Now, how do we integrate these C naming conventions into Excel? Here are steps and considerations:
Create a List of Variables

- Create a new Excel worksheet titled “Naming Conventions”.
- In column A, list your variables or function names.
- Use column B to note the type (variable, function, constant, etc.).
- In column C, describe the purpose or scope of the item.
- Format cells to highlight conventions (e.g., color coding or applying conditional formatting).
Example Table

Name | Type | Purpose |
---|---|---|
userDataCount | Variable | Counts user data entries |
calculateTotalSum | Function | Performs sum calculation |
MAX_BUFFER_SIZE | Constant | Defines maximum buffer size |

Tips for Effective Naming in Excel
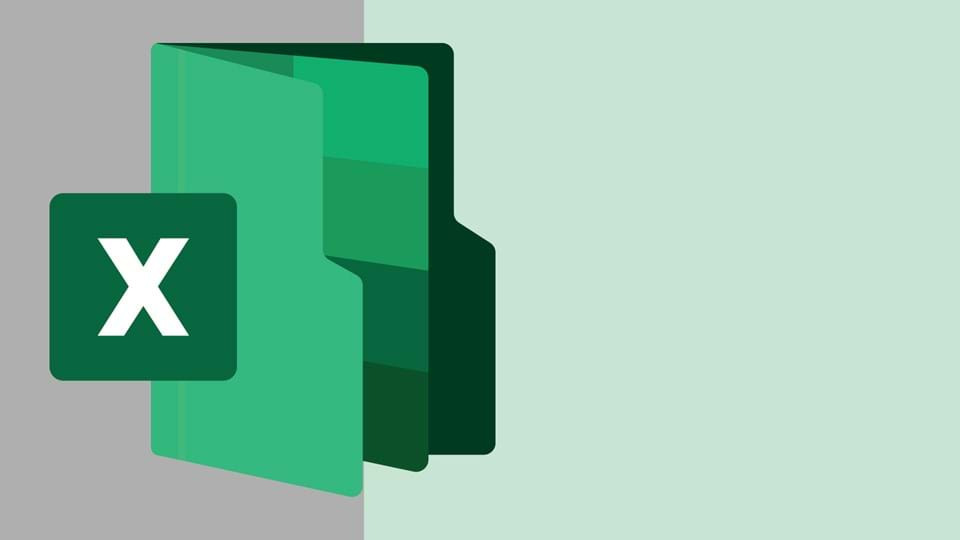
Here are some tips to ensure your Excel workbook is optimized for C naming conventions:
- Sort your list alphabetically by type for easier review.
- Use data validation to ensure names conform to conventions.
- Create categories within Excel using the grouping feature or by inserting new sheets.
💡 Note: Use Excel's naming feature for your sheets. For example, name sheets after variable categories like "Variables", "Functions", etc.
Throughout this exploration of C naming conventions in Excel sheets, we've outlined how to effectively organize and manage names in a programming context. By applying these conventions, developers can ensure their code is more readable, self-documenting, and maintainable. This practice, when integrated into tools like Excel, can also serve as a live documentation, aiding in code reviews, maintenance, and collaborative projects. Remember, consistency is key in any programming task, and by using Excel, you've now equipped yourself with a powerful tool to enforce and track naming conventions in your C projects.
Why are naming conventions important in C?

+
Naming conventions in C programming improve code readability, reduce errors in code reuse, and make maintenance and collaboration easier.
Can I use abbreviations in my variable names?

+
Yes, but only if they are widely recognized or standardized in your codebase to avoid confusion.
How do I handle global variables in naming?

+
Prefix global variables with a ‘g’ or another distinctive character to indicate their global scope, making them easier to spot in your code.