Overwrite Excel Sheets with Python Openpyxl Easily

In this post, we'll delve into how to easily overwrite Excel sheets using Python's openpyxl
library. Whether you're maintaining data logs, creating reports, or handling repetitive tasks, mastering this technique will streamline your workflow significantly.
Understanding Openpyxl

Openpyxl is a Python library designed for reading, writing, and modifying Excel 2010 xlsx/xlsm/xltx/xltm files. It’s an excellent tool for automating tasks in Excel without the need to open the software manually.
- Allows read/write access to cells, formulas, styles, etc.
- Supports multiple worksheets
- Handles large datasets efficiently
Setting up Your Environment

To start, ensure you have Python installed and openpyxl library. Here’s how you can install it:
pip install openpyxl
💡 Note: Make sure you have the necessary permissions to install packages in your Python environment.
Loading an Existing Excel File

Before you can overwrite any sheets, you’ll need to load an existing workbook:
from openpyxl import load_workbook
wb = load_workbook(‘example.xlsx’)
Overwriting Sheets

Here’s a step-by-step guide to overwrite existing sheets:
- Access the Workbook: You’ve already loaded the workbook with the above code.
- Select the Sheet: Use
wb.active
or reference it directly by name.# Accessing the active sheet sheet = wb.active
sheet = wb[‘Sheet1’]
- Clear Existing Content: If you want to replace the entire content of the sheet, clear it first.
sheet.delete_rows(1, sheet.max_row)
- Add New Content: Now, you can write new content or paste data from another source.
data = [ [‘Name’, ‘Age’, ‘Gender’], [‘John Doe’, 29, ‘Male’], [‘Jane Smith’, 25, ‘Female’] ]
for row in data: sheet.append(row)
- Save Changes:
wb.save(‘example.xlsx’)
Creating or Replacing Sheets

If you want to create a new sheet or overwrite an existing one:
- To create a new sheet:
wb.create_sheet(‘New Sheet’)
- To overwrite an existing sheet with new data:
if ‘Old Sheet’ in wb.sheetnames: wb.remove(wb[‘Old Sheet’])
wb.create_sheet(‘New Sheet’, 0) # Index 0 places the new sheet at the beginning
Multiple Sheets

Handling multiple sheets can be done as follows:
Action | Python Code |
---|---|
Add a New Sheet | wb.create_sheet(‘SheetName’) |
Remove a Sheet | wb.remove(wb[‘SheetName’]) |
Access a Specific Sheet | sheet = wb[‘SheetName’] |
Iterate Through Sheets | for sheet in wb.worksheets:
print(sheet.title) |

Finalizing Changes

After making all the necessary changes to your workbook, don’t forget to:
- Save the workbook to apply all changes.
- Ensure you’ve saved in the correct file format, especially if you’re dealing with macros or compatibility issues.
wb.save(‘example.xlsx’)
📌 Note: Remember to use .xlsx
for standard Excel files. If you’re working with macros or need compatibility with older Excel versions, consider saving as .xlsm
or .xls
.
To wrap up, by mastering the process of overwriting Excel sheets with Python and openpyxl
, you can automate complex data manipulations and reporting tasks with ease. This skill not only saves time but also reduces the likelihood of human error, making your data management more robust. The approach we’ve covered allows you to create or modify Excel files programmatically, providing a powerful tool for data analysts, researchers, and anyone dealing with Excel-based tasks.
Can I use openpyxl to edit formulas?

+
Yes, openpyxl supports editing cell formulas. You can use cell.value = ‘=SUM(A1:A5)’
to apply a formula to a cell.
How do I overwrite a cell’s value with openpyxl?

+
To overwrite a cell’s value, access the cell and set its value like this: sheet[‘A1’].value = ‘New Value’
.
What if I need to work with multiple workbooks?
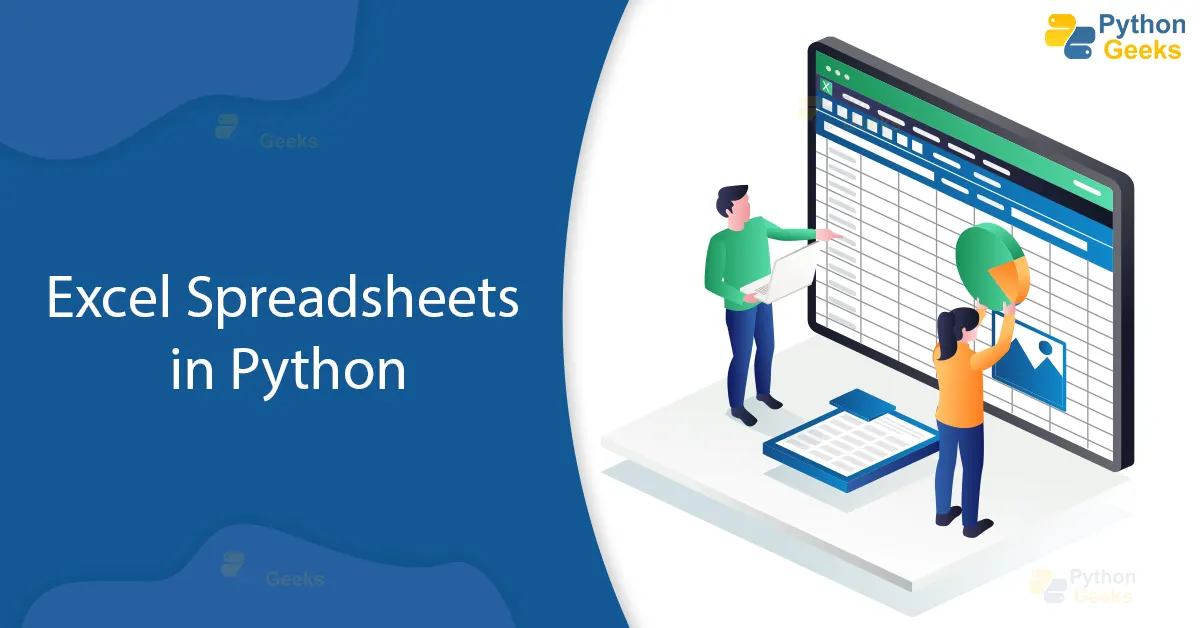
+
You can work with multiple workbooks by loading each with load_workbook()
. You can then copy data from one to another, but remember to save each workbook individually.